C++ is the best and extensively used programming language for creating video video games.
It’s additionally the language Unreal Engine, arguably the preferred sport engine on this planet, makes use of too.
Nonetheless, there are some variations between the normal C++ programming software program builders use and C++ that’s utilized in Unreal Engine to create video video games. The ideas are the identical, simply the syntax is barely totally different.
On this submit we’ll cowl in depth the variations between C++ for software program growth and C++ for Unreal Engine sport growth.
Earlier than we begin, just be sure you obtain Unreal Engine and Visible Studio. You possibly can observe the information within the hyperlinks beneath to do this:
Obtain Unreal Engine And Taking A Look At Its Interface
For each instance we use, first we’ll present the way it’s completed in conventional C++ software program growth language, then we’ll present the identical instance the way it’s completed with C++ in Unreal Engine.
/* widget: Random Picture – Center */
#uc_ue_random_image_elementor_36cae1d913
{
show:flex;
}
#uc_ue_random_image_elementor_36cae1d913 .ue-image-item
{
show:block;
transition:0.3s;
width:100%;
}
#uc_ue_random_image_elementor_36cae1d913 .ue-image-link
{
show:block;
coloration: unset;
}
Variables In C++
Variables are the center of any software program software or online game. We use them to declare the properties within the app or sport which is able to signify the excessive rating, well being, pace, ammo rely, names of sport characters, and so forth.
Which means there are various kinds of variables corresponding to entire numbers, decimal level numbers, strings, and booleans.
That is how we declare them in pure C++:
// this embrace will add the library e.g. predefined
// code that features the string variable
#embrace ;
// the title area std is the place the string is outlined
utilizing namespace std;
class Participant
{
int Well being = 100;
float Pace = 5.5f;
string Title = "Wizard";
bool IsDead = true;
};
When declaring a variable, first we declare its kind, then we give it a reputation, then we give it an non-compulsory worth and we finish the declaration usimg a semi colon ” ; “.
The kind of variable is represented by int, float, string, and bool. We’ll speak about each variable kind in second.
And the worth of that variable is represented by the worth we add after the non-compulsory ” = ” signal. We are saying non-compulsory as a result of we are able to additionally declare a variable like this:
int Well being;
This implies we declared a variable of kind int, with the title Well being and no worth. After all, each variable has a default worth, which we’ll speak about as effectively.
And we are able to all the time change the values of any variable we declare therefore why they’re referred to as variables. Now let’s go line by line very variable we declared and clarify what it’s.
The variables are declared on strains 10, 11, 12, and 13.
Variable declared on line 10 is named an integer, or quick int. Integers are entire numbers that means they’ll’t have decimal factors of their worth.
A float declared on line 11 then again is a decimal level quantity.
And even for those who declare a float worth which is a complete quantity, you continue to have to incorporate a decimal level by including at the least the dot ” . “:
// this may present an error in code
float Pace = 5f;
// that is the right solution to declare a complete float
float Pace = 5.0f;
// that is the right solution to declare a complete float
float Pace = 5.f;
The variables declared on strains 2 and 5 are precisely the identical and could have the identical worth. They’re each float with the worth of 5. The one distinction is after we declared the one on line 5, we included the decimal worth.
One factor you’ll discover with float variables is that we add ” f ” on the finish of the declaration. The explanation why we add ” f ” on the finish of a float declaration is as a result of we have now one other decimal level kind variable referred to as a double.
That is how we declare it:
double Mana = 2.4;
The distinction between a float and a double is within the precision. A double is extra exact than a float, however these precisions go within the excessive decimal level values.
An instance for that will be a quantity like this:
3.19827396781623871628376
However there’s nearly no case in sport growth the place you’ll want a decimal quantity that has greater than 3 decimal factors.
We’re working within the sport dev business since 2015 and we solely encountered a decimal quantity with 2 factors at most throughout our profession.
And that’s why float is used greater than a double in sport growth and no sport engine is an exception to this.
Subsequent, we have now a string variable declared on line 12 within the above instance. Strings signify characters and we use them to create variables that typically will repsent the names or tags of sport objects.
Additionally, once you declare a price of a string, that you must use quotes (“”):
string Title = "Wizard";
And the final variable declared on line 13 within the instance above is a boolean or quick bool.
Whereas all variabels we noticed to this point can have any quantity worth or character worth for a string, booleans can solely have one of many two values: true or false.
The IsDead bool worth above has a price of true. We will additionally declare the worth of that variable to be false, and that’s it.
Booleans are used for conditional statements and for evaluating variables with each other. We’ll see examples of this as effectively.
Now, let’s check out how can we declare these similar variables inside Unreal Engine and the syntax differerence between C++ for Unreal, and C++ for software program growth.
C++ Variables In Unreal Engine
As we already talked about, C++ for software program growth and C++ for Unreal Engine are one and the identical language. The ideas and the rules are the identical, the one distinction is the syntax.
And syntax means the best way how the code is written. So let’s check out that distinction.
Right here’s how we declare the identical sorts of variables we noticed within the instance above inside a C++ class in Unreal Engine:
int Well being = 100;
float Pace = 5.5f;
FString Title = "Wizard";
bool IsDead = false;
As you may see all of the variables precisely the identical aside from the string variable which is known as FString.
Except for the title being totally different e.g. the string inside Unreal Engine has F in entrance of it, every little thing else is similar.
The FString variable capabilities the identical approach because the string variable inside C++. We nonetheless must put the worth of that string between quotes “” and it’s a personality kind variable.
And that is that syntax distinction. Basically you’re utilizing the identical factor, however you’re writing it another way, that’s it.
We will additionally delcare a double the identical approach we did in C++ within the instance above:
double Mana = 75.5;
Once more, we’ll use floats more often than not inside Unreal Engine, however that is simply to point out you that these variables are the identical in Unreal Engine in addition to C++.
Math Operations With Variables
We will carry out math operations with variables. That is how we transfer the characters, shoot bullets, rely rating and lots of different issues in our video games.
For some motive, lots of people suppose that that you must be a math genious to do that, however as you’ll see in a second, all that you must know is fundamental math operations like addition, subtraction, multiplication and division.
Right here’s how that appears like in C++:
#embrace
int primary()
{
float Well being = 55.0f;
float HealAmount = 10.0f;
float Injury = 5.0f;
std::cout << "The remaining well being is: " << Well being + HealAmount;
std::cout << "nThe remaining well being is: " << Well being - Injury;
std::cout << "nThe remaining well being is: " << Well being * HealAmount;
std::cout << "nThe remaining well being is: " << Well being / Injury;
}
After we run this program that is the output we get within the console:
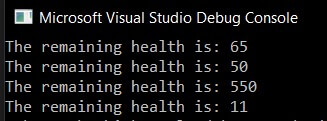
These are the fundamental math calculations we have to know to create video games in Unreal Engine.
You would wish to know superior math operations solely if you wish to work as a graphics developer, physics engine developre, or work on vector math. In any other case, these fundamental operations are sufficient to create just about any sport you need inside Unreal Engine.
And talking of Unreal Engine, let’s see how these similar math operations appear like inside Unreal:
void APlayerCharacter::BeginPlay()
{
Tremendous::BeginPlay();
float Well being = 55.0f;
float HealAmount = 10.0f;
float Injury = 5.0f;
float HealthAddition = Well being + HealAmount;
float HealthSubtraction = Well being - Injury;
float HealthMultiplication = Well being * HealAmount;
float HealthDivision = Well being / Injury;
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Addition is: %f"), HealthAddition);
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Subtraction is: %f"), HealthSubtraction);
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Multiplication is: %f"), HealthMultiplication);
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Division is: %f"), HealthDivision);
}
After we run the sport that is the out we get within the Output Log tab:

By performing the identical operations on the identical values we bought the identical end result each in C++ uncooked software and in Unreal Engine.
The one distinction is that in Unreal Engine it shows six decimal level numbers, however don’t fear about that since that’s the default approach how the output works and we are able to after all restrict this to 5, 4, even one decimal level quantity. However since that’s not the purpose of this submit we is not going to dive into that matter.
/* widget: Random Picture – Center */
#uc_ue_random_image_elementor_36cae1d914
{
show:flex;
}
#uc_ue_random_image_elementor_36cae1d914 .ue-image-item
{
show:block;
transition:0.3s;
width:100%;
}
#uc_ue_random_image_elementor_36cae1d914 .ue-image-link
{
show:block;
coloration: unset;
}
Capabilities In C++
In each program or online game we create we’ll have code that we reuse again and again.
And if that code has let’s say 100 strains, as an alternative of copy-pasting these 100 strains in each a part of the undertaking the place we want it, we are able to group that code in a operate and reuse it every time we want it.
Utilizing the maths operations with variables instance, we’re going to create capabilities for each math operation.
Right here’s how that appears like in C++ software:
#embrace
int primary()
{
AddNumbers(55.0f, 10.0f);
SubtractNumbers(55.0f, 5.0f);
MultiplyNumbers(55.0f, 10.0f);
DivideNumbers(55.0f, 5.0f);
}
float AddNumbers(float a, float b)
{
std::cout << "The Addition worth of the numbers is: " << a + b;
}
float SubtractNumbers(float a, float b)
{
std::cout << "The Subtraction worth of the numbers is: " << a - b;
}
float MultiplyNumbers(float a, float b)
{
std::cout << "The Multiplication worth of the numbers is: " << a * b;
}
float DivideNumbers(float a, float b)
{
std::cout << "The Division worth of the numbers is: " << a / b;
}
After we run this system that is the output we get within the console:
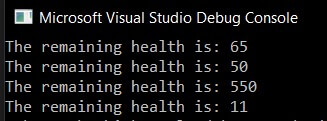
We principally took the identical operations from the earlier instance, put them in a operate and now we are able to merely go two float numbers as parameters that we’ll use contained in the operate to carry out the precise operation.
The capabilities we declare return a float worth and take two parameters. The worth that’s being returned in addition to the parameter values are a float kind.
After all, we are able to set these values to be integers, booleans, or strings.
You possibly can study in-depth about capabilities by clicking on the hyperlink beneath:
Now let’s check out how can we declare the identical capabilities inside Unreal Engine:
float AddNumbers(float a, float b);
float SubtractNumbers(float a, float b);
float MultiplyNumbers(float a, float b);
float DivideNumbers(float a, float b);
First, we declare the capabilities contained in the .h file of the C++ class inside Unreal Engine. Basically, in Unreal Engine once you create a C++ class mechanically you get a .h and .cpp file for that class.
Within the .h file you declare all of the variables and capabilities for that class, and contained in the .cpp file you implement the performance of the variables and capabilities.
It is a temporary rationalization as we’ll not dive into that matter greater than that for this submit since we already produce other posts the place we clarify that in-depth.
We’ll put hyperlinks of these posts on the backside of this one and you’ll test them out after you end this submit.
After we declared the capabilities within the .h file, we implement them within the .cpp file:
void APlayerCharacter::BeginPlay()
{
Tremendous::BeginPlay();
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Addition is: %f"), AddNumbers(55.0f, 10.0f));
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Subtraction is: %f"), SubtractNumbers(55.0f, 5.0f));
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Multiplication is: %f"), MultiplyNumbers(55.0f, 10.0f));
UE_LOG(LogTemp, Warning, TEXT("The worth of well being Division is: %f"), DivideNumbers(55.0f, 5.0f));
}
float APlayerCharacter::AddNumbers(float a, float b)
{
return a + b;
}
float APlayerCharacter::SubtractNumbers(float a, float b)
{
return a - b;
}
float APlayerCharacter::MultiplyNumbers(float a, float b)
{
return a * b;
}
float APlayerCharacter::DivideNumbers(float a, float b)
{
return a / b;
}
The APlayerCharacter is the title of the category which we use after we implement the operate performance like this:
return worth ClassName::FunctionName(parameters if any)
{
implementation of the operate
}
After we run the sport that is the out we get within the Output Log tab:

Since we used the identical numbers, the values are precisely the identical as within the C++ instance and within the earlier Unreal Engine instance.
Conditional Statements
In programming and sport growth we all the time have conditions the place selections are made.
Must you press the left or the correct button, does the participant must go left or proper, is the well being worth decrease or better than zero and so forth.
In programming and sport growth we use conditional statements to create selections within the software or sport.
That is how a conditional assertion seems like inside C++ app:
if (true)
{
// code goes right here
}
else
{
// code goes right here
}
Conditional statements are additionally referred to as if else statements. The best way if else statements work is you check for a situation, and if that situation is true the clause the place that situation is true will probably be executed.
Within the instance above, the caluse within the if(true) will probably be executed and the clause for the else is not going to be executed.
One factor you’ll discover is the “true” a part of the if else assertion. That is the place booleans come into play.
For those who keep in mind in the beginning of the submit after we talked concerning the bool variable we stated we use it to check circumstances in our initiatives, and that is how that appears like.
After all, when writing an app or a sport we’ll by no means use if(true) for an announcement, as an alternative we’d have variables that point out a state within the sport, corresponding to IsAlive:
bool IsAlive = true;
if (IsAlive)
{
// participant continues to be alive do not finish the sport
}
else
{
// participant is useless. finish the sport
}
Within the instance above the bool declared on line 1 will all the time have a price of true, however in a sport occasions will occur that may decrease the participant’s well being worth to or beneath zero after which the IsAlive variable could have a price of false after which we are able to run the sport over code.
We will additionally examine numbers with if else statements:
float a = 10.0f;
float b = 5.0f;
if (a > b)
{
// a is larger than b
}
else
{
// a is lower than b
}
Once more, within the instance above a and b will all the time have a price of 10 and 5, however that is simply to exhibit how the if else statements work.
In an actual undertaking the values you declare to your lessons will all the time change and thus the comparisons you make will probably be dynamic.
Listed here are all of the comparisons we are able to make with numbers:
float a = 10.0f;
float b = 5.0f;
if (a > b)
{
// a is larger than b
}
if (a >= b)
{
// a is larger than or equal to b
}
if (a < b)
{
// a is lower than b
}
if (a <= b)
{
// a is lower than or equal to b
}
if (a == b)
{
// a is the same as b
}
And right here’s one other approach how we are able to write these similar circumstances:
float a = 10.0f;
float b = 5.0f;
if (a > b)
{
// a is larger than b
}
else if (a >= b)
{
// a is larger than or equal to b
}
else if (a < b)
{
// a is lower than b
}
else if (a <= b)
{
// a is lower than or equal to b
}
else if (a == b)
{
// a is the same as b
}
/* widget: Random Picture – Center */
#uc_ue_random_image_elementor_36cae1d915
{
show:flex;
}
#uc_ue_random_image_elementor_36cae1d915 .ue-image-item
{
show:block;
transition:0.3s;
width:100%;
}
#uc_ue_random_image_elementor_36cae1d915 .ue-image-link
{
show:block;
coloration: unset;
}
Discover within the instance above we’re utilizing else if statements and within the instance previous to that we had been simply utilizing if statements.
One other factor to level out is for the final comparability if a is the same as b. We’re utilizing the double equal signal ” == ” to match the 2 numbers.
So once you’re evaluating two numbers if their values are equal you employ the double equal signal ” == ” however once you declare a price for a variable you then use a single equal signal ” = “.
On this case, there’s no distinction within the end result we get for the circumstances we’re testing between the if statements and if else statements, however we are able to use totally different conditional assertion constructions to check totally different circumstances.
For instance:
float a = 10.0f;
float b = 5.0f;
if (a > b)
{
// a is larger than b
}
else if (a < b)
{
// a is lower than b
}
else
{
// a is neither better than or lower than b
}
Within the instance above, if a is larger than b we’ll execute the code for that situation. If a is lower than b we’ll execute the code for that situation. And lastly, if neither of these circumstances is true, we’ll execute the code within the “else” clause.
In a sensible instance this might appear like this:
if (wolf enemy assaults the participant)
{
// deal 10 injury to the participant
}
else if (hawk enemy assaults the participant)
{
// deal 20 injury to the participant
}
else
{
// deal 5 injury to the participant
}
After all, in our sport we’d not title the variables like within the instance above, however that is simply as an instance totally different conditions we would have in a sport.
One other factor to notice is that each one these comparisons find yourself being both true or false, therefore the booleans involment in conditional statements.
After we check if a > b this may both lead to true if a is larger than b, or false if a isn’t better than b.
This additionally signifies that we are able to set boolean values like this:
float a = 5.0f;
float b = 4.0f;
bool c = a > b;
We’re evaluating if variable a is larger than b which is able to lead to both true or false, and we set that worth to the boolean c variable.
After all, within the instance above the worth will probably be true, so if we had been to print the worth of the c variable we’d see true being printed within the console.
Now let’s go over to Unreal Engine and see how the conditional statements look inside Unreal Engine:
float a = 5.5f;
float b = 3.3f;
if (a > b)
{
// a is larger than b
}
else if (a b)
{
// a is larger than b
}
if (a = b)
{
// a is larger than or equal to b
}
if (a <= b)
{
// a is lower than or equal to b
}
if (a == b)
{
// a is the same as b
}
As you may see there’s no distinction between utilizing conditional statements in a C++ software or Unreal Engine sport.
C++ Loops
One other factor that we do usually is apps and video video games is repeat a sure mechanic. And we try this with the assistance of loops.
That is how a loop seems like in C++ app:
#embrace
int primary()
{
for (int i = 0; i < 10; i++)
{
std::cout << "The worth of i is: " << i << "n";
}
}
After we run the app, that is what we’ll see printed within the console:
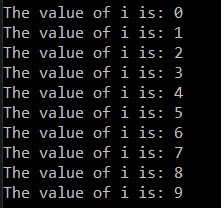
The loop within the instance above is named a for loop. And the for loop principally loops over the code that’s between the curly brackets ” {} “, within the instance above it’s calling the std::cout code 10 occasions.
Why 10 occasions?
As a result of the situation of the loop is i < 10, which suggests it is going to loop for so long as i has a price that’s lower than 10. Then it is going to increment the i worth by 1 utilizing the ++ syntax which principally means take the present worth if i and add 1 to it.
As quickly because the i worth reaches a price that’s not lower than 10, the loop will cease looping.
Right here’s a extra in-depth information about loops in C++:
Now we’re going to check out how a for loop seems like inside Unreal Engine:
void APlayerCharacter::BeginPlay()
{
Tremendous::BeginPlay();
for (int i = 0; i < 10; i++)
{
UE_LOG(LogTemp, Warning, TEXT("The worth i is: %i"), i);
}
}
After we run the sport, that is what we’ll see printed within the Output Log tab:
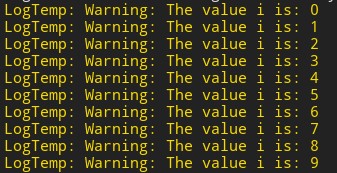
As you may see the C++ for loop is strictly the identical in Unreal Engine as effectively. And the identical explanations are legitimate for each variations.
Let’s check out one other kind of loop referred to as some time loop.
Right here’s a C++ instance of the whereas loop:
whereas(situation is true)
{
// execute the code
}
That is how the construction from the for loop would appear like shortly loop:
int i = 0;
whereas(i < 10)
{
std::cout << "The worth if i is " << i << "n";
i++;
}
After we run the app, that is what we’ll see within the console:
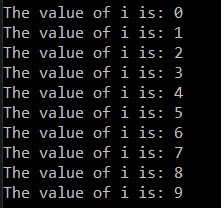
Whereas the construction is barely totally different, the aim and use are the identical.
The one factor we should be cautious of with the whereas loop is to make the situation of the loop finally be false, in any other case, we’d have an infinite whereas loop.
You possibly can learn extra about that within the hyperlink beneath:
And right here’s how some time loop seems like in Unreal Engine:
void APlayerCharacter::BeginPlay()
{
Tremendous::BeginPlay();
int i = 0;
whereas (i < 10)
{
UE_LOG(LogTemp, Warning, TEXT("The worth i is: %i"), i);
i++;
}
}
And after we run the sport, that is what we’ll see within the Output Log tab:
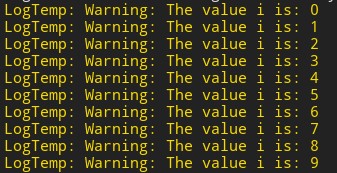
Once more, the construction and the reasons are precisely the identical. And also you additionally should be cautious concerning the whereas loop situation finally turning into false so that you just don’t have an infinite whereas loop.
/* widget: Random Picture – Center */
#uc_ue_random_image_elementor_36cae1d916
{
show:flex;
}
#uc_ue_random_image_elementor_36cae1d916 .ue-image-item
{
show:block;
transition:0.3s;
width:100%;
}
#uc_ue_random_image_elementor_36cae1d916 .ue-image-link
{
show:block;
coloration: unset;
}
Arrays In C++
The variables we noticed to this point can solely retailer one worth at a time. That is the place arrays come into play.
Arrays can retailer a number of values on the similar time and we entry these values utilizing their index quantity.
Any variable might be an array, be that integer, float, boolean, or a string. However the array can solely retailer the values of the identical variable kind.
For instance, if we create an array of integers, we are able to solely retailer integers in that array. We will’t retailer floats, strings, or booleans.
We already coated C++ arrays in one other weblog submit which you’ll take a look at by clicking the hyperlink beneath:
Utilizing the identical instance from the submit within the hyperlink above, that is how arrays are declared in C++:
int DamagePoints[10];
// FIRST WE INITIALIZE ARRAY VALUES
for (int i = 0; i < std::measurement(DamagePoints); i++)
{
DamagePoints[i] = rand();
}
// THEN WE PRINT THOSE VALUES
for (int i = 0; i < std::measurement(DamagePoints); i++)
{
std::cout << "nThe worth of ingredient is: " << DamagePoints[i];
}
And after we run the app we’ll see random integer values printed within the console.
Now, let’s do the identical in Unreal Engine:
TArray DamagePoints;
First, contained in the .h file of the C++ class we declare the array. For that we use TArray and between the <> we declare the kind of variable this array will retailer, on this case an integer.
And that is how we initialize the array:
void APlayerCharacter::BeginPlay()
{
Tremendous::BeginPlay();
for (int i = 0; i < 10; i++)
{
DamagePoints.Add(FMath::RandRange(1, 100));
}
for (int i = 0; i < DamagePoints.Num(); i++)
{
UE_LOG(LogTemp, Warning, TEXT("The worth Aspect at index %i is: %i"), i, DamagePoints[i]);
}
}
The distinction between the C++ array we noticed within the instance above and the TArray in Unreal Engine is that the TArray is a dynamic array.
Dynamic that means it’s resizeble and never fastened in measurement like the conventional C++ array. Which suggests we are able to add and take away as many parts as we wish.
However the important use is similar.
We will retailer a number of parts contained in the array, and we are able to use their indexes to entry these parts which we noticed within the examples above.
Once more, you may learn extra about arrays within the submit hyperlink beneath:
And we additionally cowl TArrays in additional depth inside Recreation Dev Professional and Recreation Dev Professional Fast Launch.
Pointers And References In C++
Each time you create a variable in your app or sport, the pc allocates reminiscence for that variable.
After all, relying on the kind of the variable the reminiscence will probably be bigger or decrease. And for those who refill the reminiscence, your app or sport will crash. That is also referred to as a reminiscence leak.
In C++, we use pointers and references to level to these spots in reminiscence that holds the variable we declared.
C++ is infamous for its pointers and references as a result of after you end utilizing them, that you must delete them or just stated that you must destroy the spots in reminiscence that maintain the variables you’re now not utilizing.
As a result of if these variables pile up within the reminiscence, you’ll get a reminiscence leak and your app or sport will crash.
This was completed manually for a few years, so builders needed to be cautious to not go away any variable once they had been completed with it in order that it doesn’t trigger this system to crash.
However through the years C++ turned higher at this and it was doing these operations mechanically.
But, loads of builders don’t know this and that’s why they’re afraid of pointers and references in C++. And they’re much more afraid of pointers and references inside Unreal Engine.
First, we’re going to check out pointers and references inside pure C++. You are able to do that by clicking on the hyperlinks beneath as a result of we already coated each matters in-depth in these posts:
Shifting ahead, now that we all know how pointers and references work in C++, let’s check out how they work inside Unreal Engine.
Assuming we have now a category referred to as PlayerCharacter, that is how we’d declare it:
APlayerCharacter* PlayerCharacter;
This decalres a variable referred to as PlayerCharacter kind of APlayerCharacter class. The ” * ” denotes that this variable is a pointer.
Since this variable is a pointer, we’ll should get rid of it after we cease utilizing it within the undertaking.
Now right here comes the nice half. Inside Unreal Engine, all we have now to do is mark that variable with the macro UPROPERTY() and this variable will probably be marked to take part within the reminiscence administration course of:
UPROPERTY()
APlayerCharacter* PlayerCharacter;
Simply by including this single line will make the GC or rubbish assortment administration get rid of the PlayerCharacter variable after we cease utilizing it within the undertaking and we don’t should do the rest.
We will additionally mark capabilities for reminiscence administration participation by utilizing the UFUNCTION() macro:
UFUNCTION()
void Assault();
UFUNCTION() has the identical impact for a operate because the UPROPERTY() has for a variable and the identical guidelines apply.
You can even use these macros to reveal variables and capabilities to blueprints as effectively.
For a extra detailed information on how C++ reminiscence administration works inside Unreal Engine learn our quick weblog submit beneath:
Courses And Objects In C++
Courses and objects are the premise of every little thing we do inside C++ and another programming language. With lessons we mannequin the habits and with objects we execute that habits.
We already coated C++ lessons in-depth in our different weblog submit which you’ll learn by clicking on the hyperlink beneath:
For those who didn’t know what are lessons and objects, the reasons within the submit above will make you a professional at that matter and can help you perceive this idea from the group up.
Now, after we know what are lessons in C++, methods to create them and use them, let’s check out methods to do the identical inside Unreal Engine.
First, once you create a category in Unreal Engine it is going to immediate you to pick out the guardian class:
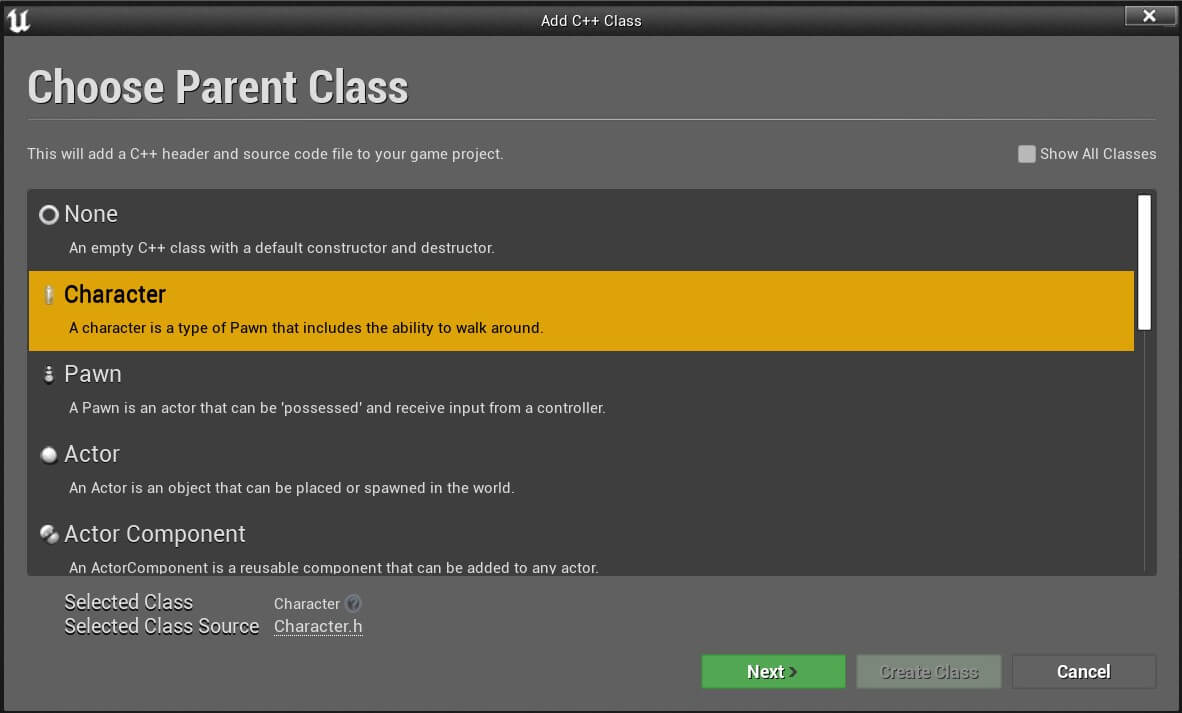
A guardian class is only a class that’s already outlined, and when a category has a guardian class that’s referred to as inheritance. We’ll cowl inheritance after the category half. However for know, simply know that in Unreal Engine you first choose the guardian class.
After you try this, it is going to take you to a different window the place you give the category a reputation and create the brand new class by urgent the Create Class button:
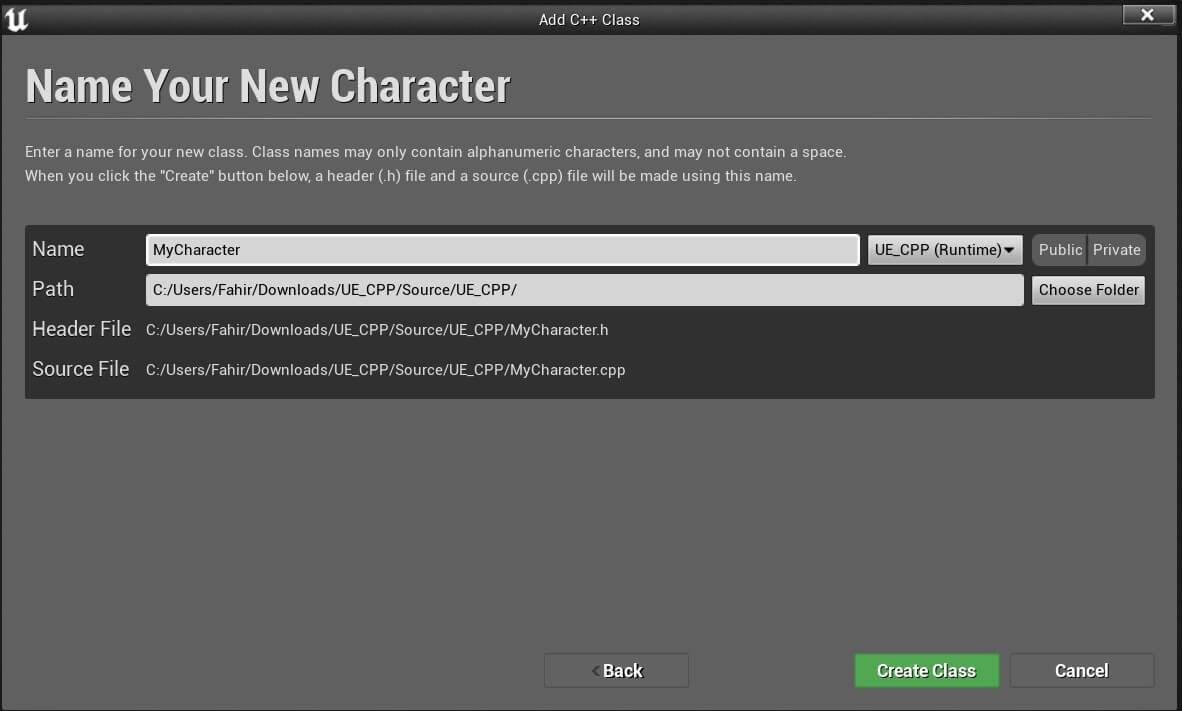
This can mechanically create two recordsdata. One .h and one .cpp file for the category.
That is the .h file of the PlayerCharacter class we created:
// Fill out your copyright discover within the Description web page of Venture Settings.
#pragma as soon as
#embrace "CoreMinimal.h"
#embrace "GameFramework/Character.h"
#embrace "PlayerCharacter.generated.h"
UCLASS()
class UE_CPP_API APlayerCharacter : public ACharacter
{
GENERATED_BODY()
public:
// Units default values for this character's properties
APlayerCharacter();
protected:
// Referred to as when the sport begins or when spawned
digital void BeginPlay() override;
public:
// Referred to as each body
digital void Tick(float DeltaTime) override;
// Referred to as to bind performance to enter
digital void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
};
And that is the .cpp file:
/* widget: Random Picture – Center */
#uc_ue_random_image_elementor_36cae1d917
{
show:flex;
}
#uc_ue_random_image_elementor_36cae1d917 .ue-image-item
{
show:block;
transition:0.3s;
width:100%;
}
#uc_ue_random_image_elementor_36cae1d917 .ue-image-link
{
show:block;
coloration: unset;
}
// Fill out your copyright discover within the Description web page of Venture Settings.
#embrace "PlayerCharacter.h"
// Units default values
APlayerCharacter::APlayerCharacter()
{
// Set this character to name Tick() each body. You possibly can flip this off to enhance efficiency for those who do not want it.
PrimaryActorTick.bCanEverTick = true;
}
// Referred to as when the sport begins or when spawned
void APlayerCharacter::BeginPlay()
{
Tremendous::BeginPlay();
}
// Referred to as each body
void APlayerCharacter::Tick(float DeltaTime)
{
Tremendous::Tick(DeltaTime);
}
// Referred to as to bind performance to enter
void APlayerCharacter::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Tremendous::SetupPlayerInputComponent(PlayerInputComponent);
}
As we already know, contained in the .h file we’ll declare all of the variables and capabilities the category could have, and contained in the .cpp file we’ll implement the performance of the category.
So if we need to declare two capabilities for the PlayerCharacter class, one referred to as Assault and the opposite referred to as Stroll, that is how we’d do it.
First, contained in the .h file:
void Assault();
void Stroll();
Then contained in the .cpp file:
void APlayerCharacter::Assault()
{
// participant assault
}
void APlayerCharacter::Stroll()
{
// participant stroll
}
As you may see, there’s no distinction how we declare and deal with lessons inside uncooked C++ and insde Unreal Engine.
After we need to use the category in one other class file, we’d additionally import the .h file of the category we need to use:
#embrace "PlayerCharacter.h"
Now as a result of in Unreal Engine we take care of actors within the stage, we don’t create objects the identical approach we did in C++ by utilizing the equals signal ” = ” as an alternative we get references to the objects.
However previous to that, we must create a reference variable contained in the .h file like this:
APlayerCharacter* PlayerCharacter;
After which, contained in the .cpp file we’d get a reference to the PlayerCharacter variable.
More often than not we get a reference to the variable when a collision occurs, or we add the reference by way of the Blueprint editor.
Since that’s a subject on it’s personal, we’ll not dive into it right here. We’ll go away hyperlinks on the backside of this submit the place you may discover ways to do that by follow by making a sport.
However for now, we’ll simply present that we are able to use the attributes of the PlayerCharacter class, the identical approach we used the attributes of the Participant class within the C++ instance:
void AClassTest::BeginPlay()
{
Tremendous::BeginPlay();
PlayerCharacter->Assault();
}
As you may see on-line 5, we’re utilizing the PlayerCharacter Assault operate and any code that’s inside that operate could be executed after we name it.
Inheritance In C++
The final a part of the foundational pillars of C++ coding is inheritance.
Inheritance principally means we are able to inherit a category we already created and outlined.
After all, we inherit a category by class. So, we create a brand new class, and inside that new class, we inherit a category we already created.
The category that inherits is named a baby class, and the category that’s being inherited is named a guardian class.
The kid class will inherit all public variables and capabilities outlined within the guardian class and it may possibly use them as its personal.
Moreover, it may possibly additionally edit the capabilities of the guardian class and alter their performance. After all, this modification will solely be utilized within the baby class, it is not going to have an effect on the guardian class.
We already coated inheritance in-depth within the submit you may learn by clicking the hyperlink beneath:
The submit above will can help you perceive every little thing about inheritance inside C++.
Now, let’s check out how inheritance works inside Unreal Engine.
After we created the PlayerCharacter class, on the very starting we had been prompted to pick out a guardian class for it.
We’re not obligated to inherit a category each time we create a brand new one inside Unreal, however a lot of the occasions the lessons we create in Unreal will inherit a predefined class corresponding to Actor, Pawn, Character and so forth.
It is because we’ll use the lessons inside the sport, and for that we’ll should inherit lessons that are already outlined that enable us to make use of our personal lessons within the sport.
After we created the PlayerCharacter class, we chosen that it ought to inherit the Character class. That is the default .h file that’s generated when the PlayerCharacter class was created:
#embrace "CoreMinimal.h"
#embrace "GameFramework/Character.h"
#embrace "PlayerCharacter.generated.h"
UCLASS()
class UE_CPP_API APlayerCharacter : public ACharacter
{
GENERATED_BODY()
public:
// Units default values for this character's properties
APlayerCharacter();
protected:
// Referred to as when the sport begins or when spawned
digital void BeginPlay() override;
public:
// Referred to as each body
digital void Tick(float DeltaTime) override;
// Referred to as to bind performance to enter
digital void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
};
On the prime on line 6, you’ll see that our APlayerCharacter class is inheriting the ACharacter class. Don’t fear concerning the A letter in entrance of the category, that’s added by default.
By inheriting the ACharacter class we additionally inherit all capabilities and variables outlined within the ACharacter class, in addition to all capabilities and variables in lessons from which ACharacter class inherited prior.
Therefore we see capabilities like BeginPlay, Tick, and SetupPlayerInputComponent.
And contained in the PlayerCharacter .cpp file, these capabilities are already outlined:
#embrace "PlayerCharacter.h"
// Units default values
APlayerCharacter::APlayerCharacter()
{
// Set this character to name Tick() each body. You possibly can flip this off to enhance efficiency for those who do not want it.
PrimaryActorTick.bCanEverTick = true;
}
// Referred to as when the sport begins or when spawned
void APlayerCharacter::BeginPlay()
{
Tremendous::BeginPlay();
}
// Referred to as each body
void APlayerCharacter::Tick(float DeltaTime)
{
Tremendous::Tick(DeltaTime);
}
// Referred to as to bind performance to enter
void APlayerCharacter::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Tremendous::SetupPlayerInputComponent(PlayerInputComponent);
}
After all, we must add the code which is able to initialize the PlayerCharacter operate and so forth, however you get the purpose which is we are able to use these capabilities contained in the PlayerCharacter class.
Once more, as you may see, inheritance is just about the identical in C++ and Unreal Engine.
And general, there’s a really small syntax distinction between uncooked C++ and C++ utilized in Unreal Engine.
The place To Go From Right here
On this submit we proved improper all those that say that the “regular” C++ is totally different from the C++ utilized in Unreal Engine, and hopefully this may encourage extra individuals to study C++ and Unreal Engine after they see that it’s not onerous in any respect for the reason that rules are precisely the identical.
To implement and see these ideas in motion, you may observe our Facet Scroller C++ sport tutorial by clicking on the hyperlink beneath:
Create a Facet Scroller C++ Recreation In Unreal Engine
You can even take a look at our flagship program referred to as Recreation Dev Professional. It should can help you grasp Unreal Engine, C++, and Blueprints, and get employed in the most effective sport studios on this planet.
It did the identical for each scholar who took motion and applied the rules we speak inside.
You possibly can test it out by clicking the hyperlink right here: Recreation Dev Professional
Aside from that, you may take a look at different Unreal Engine weblog posts we have now by clicking on the hyperlink beneath:
We publish new posts on a regular basis as a result of our aim is to have a platform the place the entire world can come to discover ways to make video games with Unreal Engine so there’s all the time one thing new to find.
/* widget: Random Picture – Center */
#uc_ue_random_image_elementor_36cae1d918
{
show:flex;
}
#uc_ue_random_image_elementor_36cae1d918 .ue-image-item
{
show:block;
transition:0.3s;
width:100%;
}
#uc_ue_random_image_elementor_36cae1d918 .ue-image-link
{
show:block;
coloration: unset;
}